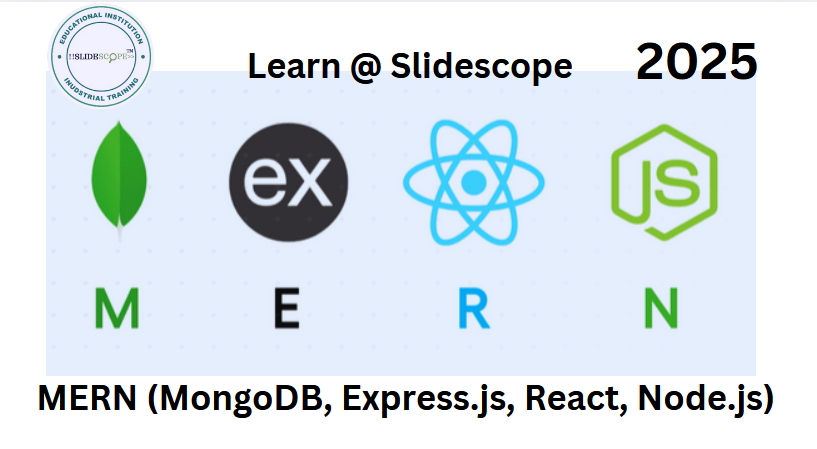
Do you want to master the skills that top companies demand and become a Full Stack MERN Developer in 2025? Let me guide you step by step on this exciting journey!
The MERN (MongoDB, Express.js, React, Node.js) stack is one of the most popular and powerful technology stacks for full-stack web development. Here’s a detailed roadmap for becoming a proficient MERN stack developer in 2025:
Phase 1: Foundation (1-2 months)
1. Learn the Basics of Web Development
- HTML: Semantic tags, forms, tables, media elements.
- CSS: Flexbox, Grid, transitions, animations, responsive design (using Media Queries).
- JavaScript:
- Variables, data types, loops, and conditions.
- DOM manipulation and Events.
- ES6+ features (let/const, arrow functions, promises, async/await, destructuring, modules).
2. Version Control & Collaboration
- Git & GitHub:
- Git basics: init, add, commit, push, pull, branches.
- GitHub: Repositories, pull requests, and collaboration.
3. Basics of Node.js
- Understand JavaScript runtime and asynchronous programming.
- Learn how to create a simple server with Node.js.
Phase 2: Backend Development (2-3 months)
1. Node.js
- Core modules: fs, path, http, events, and streams.
- Learn npm for dependency management.
2. Express.js
- Create routes and middleware.
- Work with query parameters and body data.
- Understand templating engines like EJS (optional).
3. Database with MongoDB
- Basics of NoSQL and MongoDB.
- CRUD operations (Create, Read, Update, Delete).
- Use Mongoose for schema creation and database interaction.
4. Authentication & Security
- Implement JWT (JSON Web Tokens) and sessions.
- Basic security measures: Helmet.js, CORS, and bcrypt for hashing passwords.
Phase 3: Frontend Development (2-3 months)
1. React.js
- Core concepts: Components, Props, State, Lifecycle methods.
- Functional components and Hooks (useState, useEffect, useContext).
- Router: React Router for navigation.
2. Styling
- Learn CSS frameworks like Bootstrap, Material-UI, or Tailwind CSS.
- Explore CSS-in-JS solutions like Styled-Components.
3. State Management
- Context API.
- Advanced: Redux or Zustand.
4. API Integration
- Fetch APIs using Axios or Fetch.
- Handle loading states and errors.
Phase 4: Full-Stack Integration (2-3 months)
1. MERN Stack
- Build projects where:
- MongoDB is used for the database.
- Express.js serves APIs.
- React.js handles the frontend.
- Node.js acts as the server.
2. Deployment
- Host applications on:
- Frontend: Vercel, Netlify.
- Backend: Render, Railway, or AWS.
- Database: MongoDB Atlas.
3. Advanced Concepts
- Real-time applications using Socket.io.
- Server-side rendering (SSR) with Next.js.
- GraphQL (alternative to REST APIs).
Phase 5: Advanced Skills (Ongoing)
1. Advanced Topics
- Dockerize your applications.
- Learn Microservices architecture.
- Add CI/CD pipelines (GitHub Actions, Jenkins).
2. Performance Optimization
- Backend: Database indexing, caching (Redis).
- Frontend: Lazy loading, React.memo, and dynamic imports.
3. Testing
- Frontend: Jest, React Testing Library.
- Backend: Mocha, Chai, Supertest.
4. Build a Portfolio
- Project Ideas:
- Blogging platform.
- E-commerce application.
- Social media dashboard.
- Real-time chat application.
Tools & Resources:
- Documentation: MongoDB, Express, React, Node.js.
- Courses:
- “The Complete Node.js Developer Course” (Udemy).
- “React – The Complete Guide” (Udemy).
- Practice Platforms: LeetCode, HackerRank (for problem-solving).
By following this roadmap and consistently building projects, you’ll be ready for full-stack MERN developer roles by 2025.